Tykätty ja tehokas taloushallinnon ohjelmisto
Selkeämpi taloushallinto – ja kaikki täsmää. Kaikki kirjanpidosta palkanlaskentaan ja laskutuksesta tilinpäätöksiin, yhdessä saumattomassa järjestelmässä.
Tilitoimiston arki on usein täynnä deadlineja. Fennoa tuo mielenrauhan taloushallinnon tehtäviin.

Palveleva
Kumppani, ei vain järjestelmä. Ymmärrämme taloushallinnon arkea ja rakennamme palvelua sen mukaan – yhdessä. Luomme ratkaisuja, jotka tukevat sujuvaa yhteistyötä kirjanpitäjän ja yrittäjän välillä. Vuosikymmenten kokemus taloushallintoalasta on mukana kaikessa, mitä teemme.

Toimiva
Fennoa on tykätty käyttäjien parissa – niin tilitoimistojen, kuin tilitoimistojen asiakkaidenkin. Fennoan auttaa taloushallinnon automatisoinnissa ja tehostamisessa, jättäen aikaa olennaiseen.

Kehittyvä
Ohjelmisto kehittyy tilitoimistojen mukana ja muuttuvat säädökset huomioidaan suoraan tilitoimistojen näkökulmasta. Olemme kotimainen toimija, jonka palvelimet sekä data sijaitsevat Suomessa ja päätökset tehdään lähellä sinua. Fennoan matkassa pysyt aina kehityksessä mukana.
Taloushallinto ajan tasalla – vähemmällä vaivalla
Fennoa automatisoi taloushallinnon rutiinit ja tekee kirjanpidosta reaaliaikaista. Tilinpäätös ja viranomaisilmoitukset syntyvät suoraan järjestelmästä – ja sinulle jää enemmän aikaa asiantuntijatyöhön.
Kirjanpito, joka toimii saumattomasti
Kaikki tarvittava tilinpäätöksistä veroilmoituksiin, yhdellä helppokäyttöisellä työkalulla. Tehokas tositekäsittely, täsmäytykset ja raportointi pitävät luvut ajan tasalla.
Tilinpäätös digitaalisesti – valmiina viranomaisille
Tilinpäätös ja veroilmoitus hoituvat Fennoassa sähköisesti alusta loppuun. Tase-erittelyt, liitetiedot ja viranomaisilmoitukset syntyvät suoraan järjestelmästä – ilman vientiä, ilman manuaalisia vaiheita.
Myyntilaskutus – tehokasta laskutusta ilman tuplatyötä
Laadi myyntilaskut, hallitse toistuvaislaskutus ja seuraa maksutilannetta – kaikki samassa järjestelmässä, joka keskustelee kirjanpidon kanssa. Kuluttaja- ja verkkolaskutuskin hoituvat vaivatta. Myyntilaskutusten luonti ja lähetys myös mobiilisti, ajasta ja paikasta riippumatta.
Ostolaskut – helppo hyväksyntä ja automaattinen käsittely
Asiakas hyväksyy ja maksaa ostolaskut parilla klikkauksella, myös mobiilissa. Sinä saat kaiken valmiina tiliöitäväksi. Älykäs automaatio ja Fennoa Äly vähentävät manuaalista työtä merkittävästi.
Maksuliikenne – maksa ilman verkkopankkia
Hoida maksut, tiliotteet ja suoritusten kohdistus suoraan Fennoasta. Ei erillisiä sopimuksia tai ohjelmia – useita pankkeja tuettuna. Tieto liikkuu reaaliajassa myös kirjanpitoon.
Palkat helposti ja oikein
Fennoassa hoidat palkanlaskennan ja viranomaisilmoitukset yhdestä paikasta.
Automatisoitu palkanlaskenta säästää aikaa ja vähentää virheitä. Hyväksynnän voi tehdä kätevästi tietokoneella tai liikkeellä ollessa mobiilisovelluksella. Hyväksynnän jälkeen palkat siirtyvät maksuun, ilmoitukset ja palkkalaskelmat lähtevät sekä kirjanpito muodostuu – kaikki yhdellä kertaa.
Matka- ja kululaskut – ajantasaisesti ilman erillistä työtä
Työntekijät voivat kirjata kulunsa ja matkalaskunsa suoraan järjestelmään, myös mobiilissa. Laskut voi tehdä heti matkan jälkeen, jolloin mitään ei unohdu! Tiedot siirtyvät sujuvasti maksettavaksi, kirjanpitoon tiliöitäväksi, ja matkakorvaukset tulorekisteriin – ja mitään ei jää matkalle.
Sujuvampaa arkea tilitoimistoille
Ohjaamo kokoaa tilitoimiston tärkeimmät tehtävät yhdelle näkymälle, jotta mitään ei unohdu.
Paikasta ja ajasta riippumatta
Yrityksen talousasiat eivät voi aina odottaa. Fennoa Mobiili helpottaa yrittäjän arkea ja vähentää unohduksia, kun tärkeät tehtävät voi hoitaa heti niiden tullessa ajankohtaisiksi – missä ja milloin tahansa.
Integraatiot osaksi ekosysteemiä
Rajapintamme mahdollistaa toimialasta riippumatta tehokkaan ja ajantasaisen tiedonsiirron. Integraatioiden avulla voit yhdistää taloushallinnon osaksi muita järjestelmiä, kuten BI-, ERP-, CRM-, työajanseuranta- ja kassajärjestelmiä.
Miksi Fennoa?
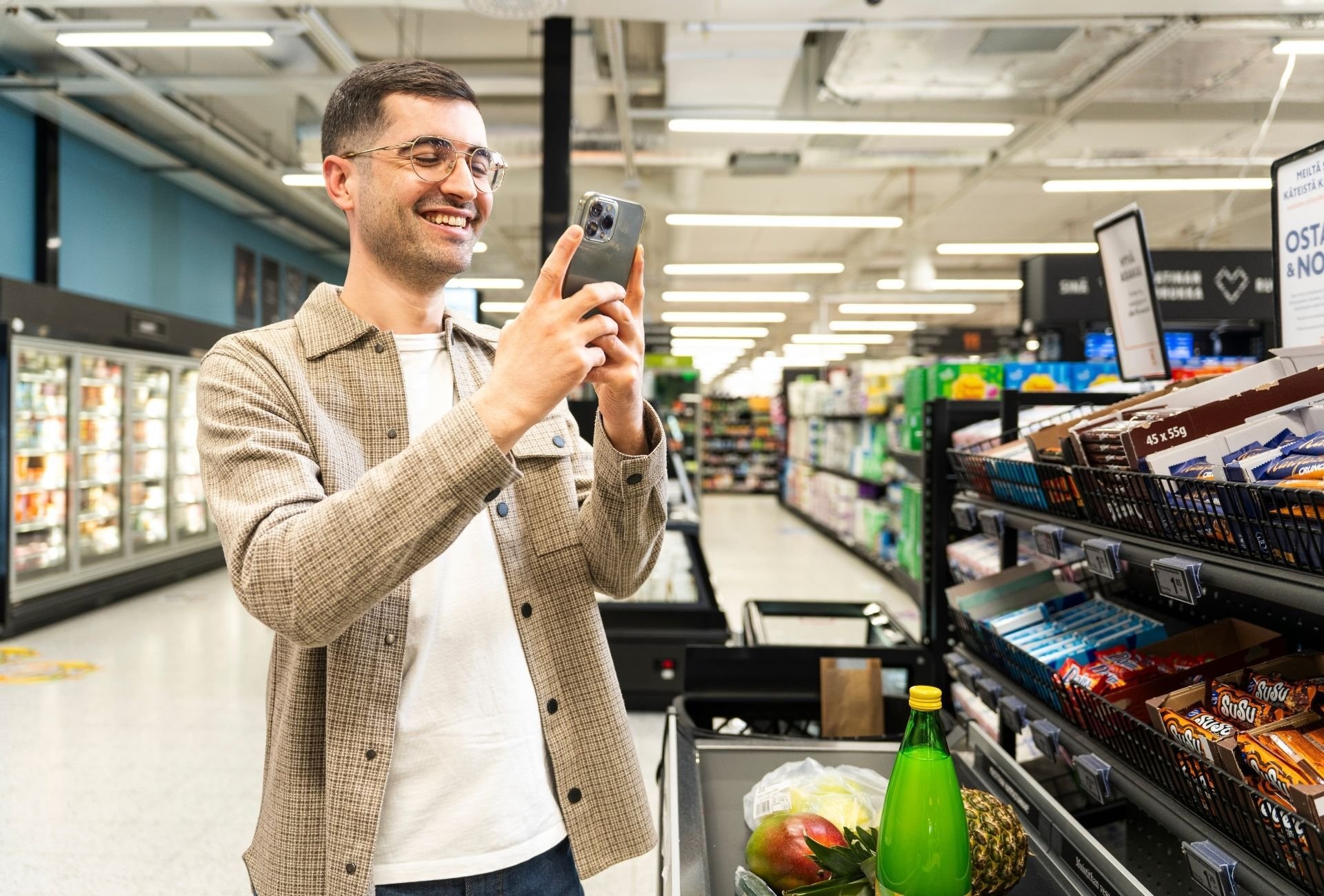
Yritykset
Kaikki taloushallintoon. Saatavilla Fennoa-tilitoimistosta.
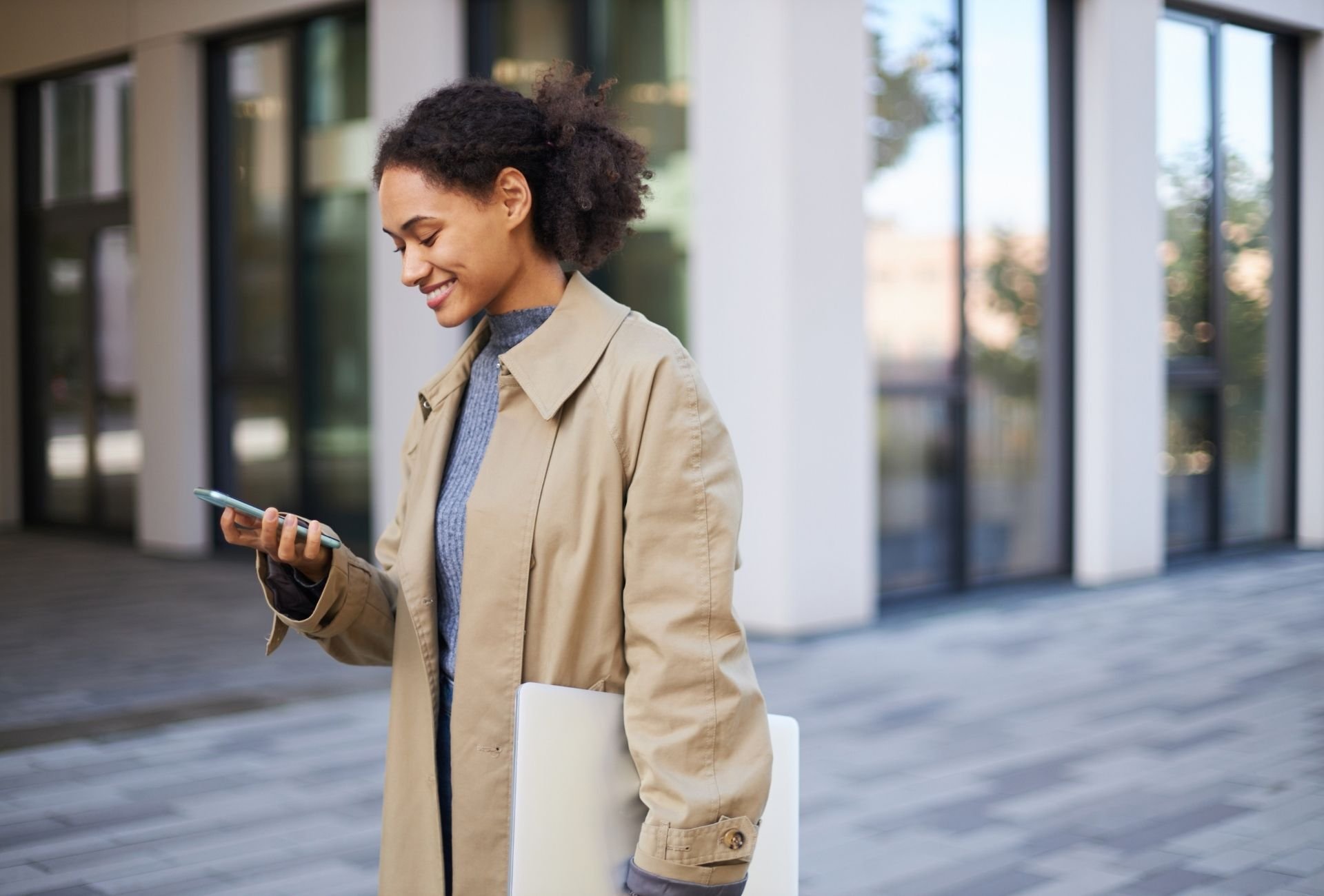
Isännöitsijät
Isännöinnin ja taloushallinnon ratkaisu yhteistyössä Avaa.io:n kanssa.
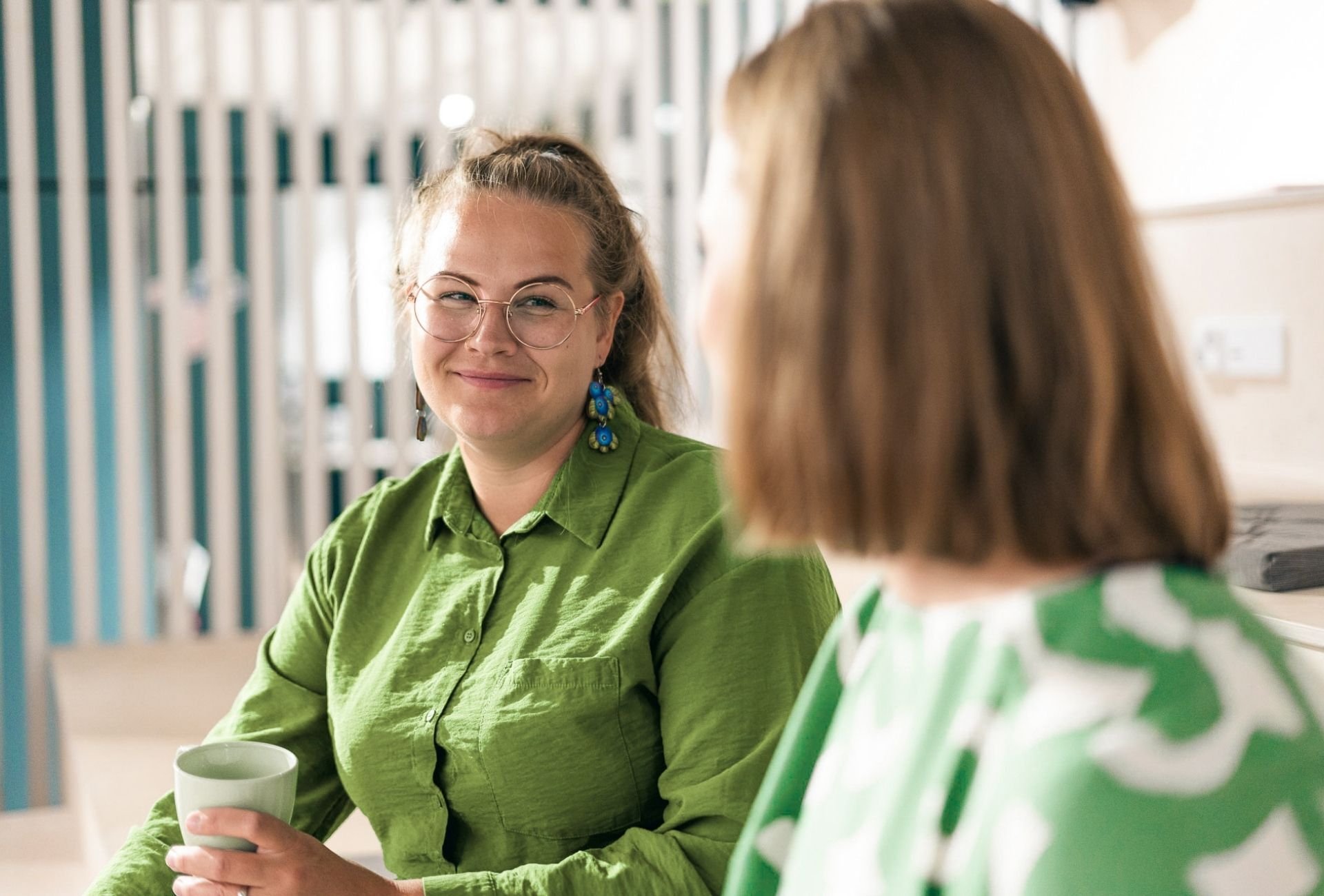
Talousosastot
Kirjanpitäjä omasta takaa? Saat Fennoan kumppanimme Softecin kautta.
Käyttäjämme suosittelevat – ja syystä. Fennoaa kehutaan erityisesti helppokäyttöisyydestä ja luotettavuudesta.
Ajankohtaista
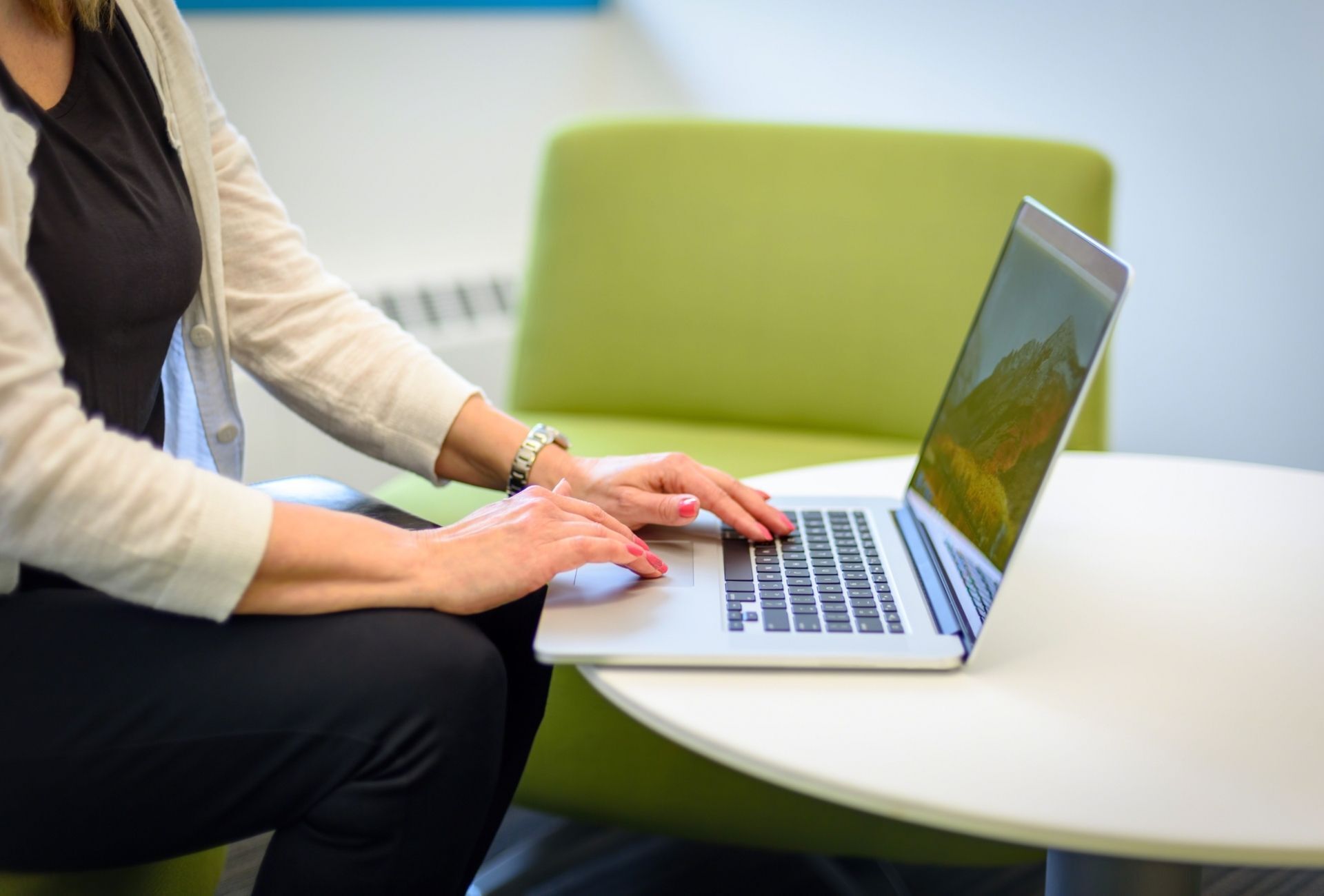
Miksi taloushallinto-ohjelmistojen hintavertailu on vaikeaa? Kirjanpitäjän vinkit pienempiin ohjelmistokuluihin
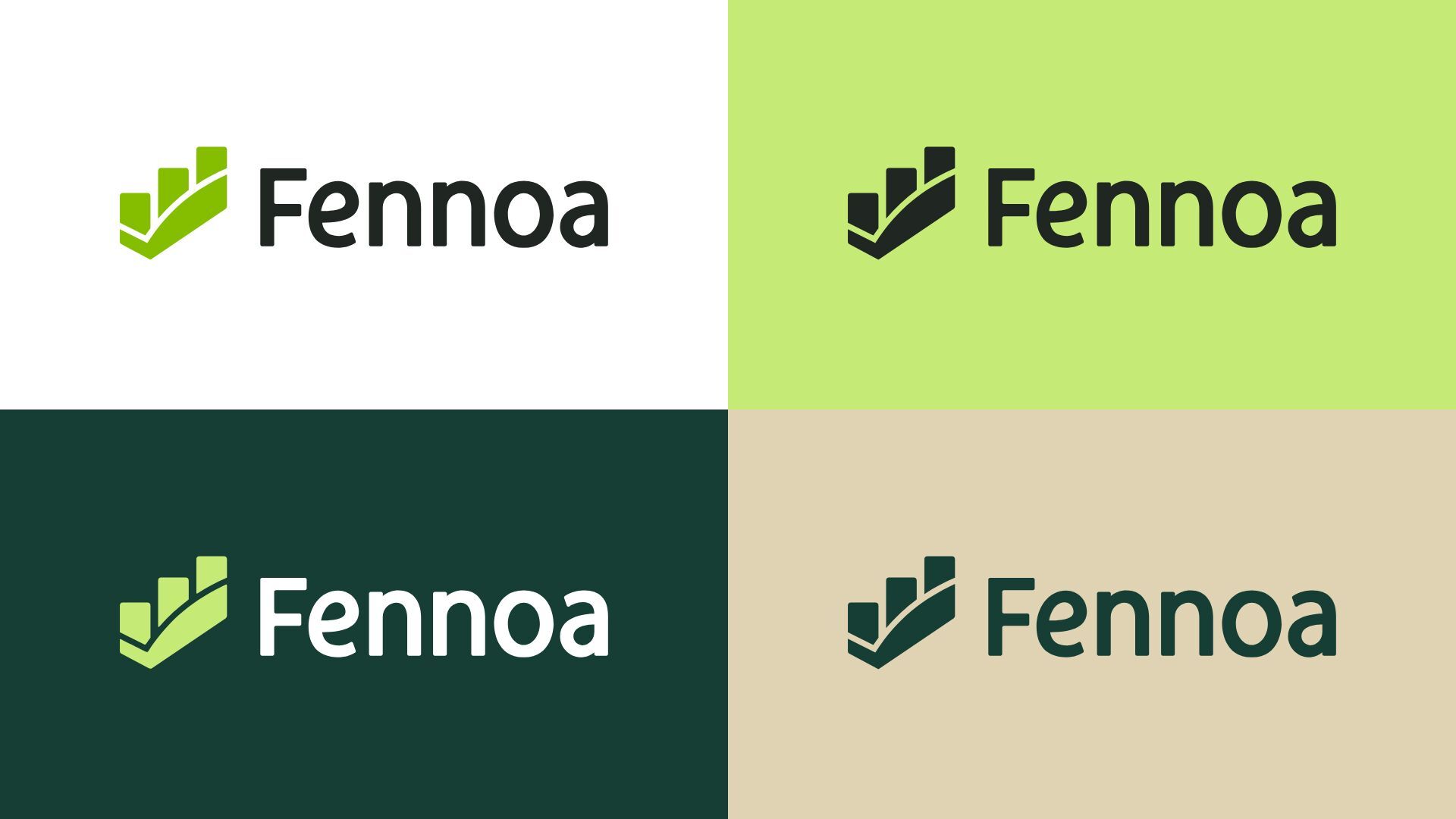
Fennoa uudistuu
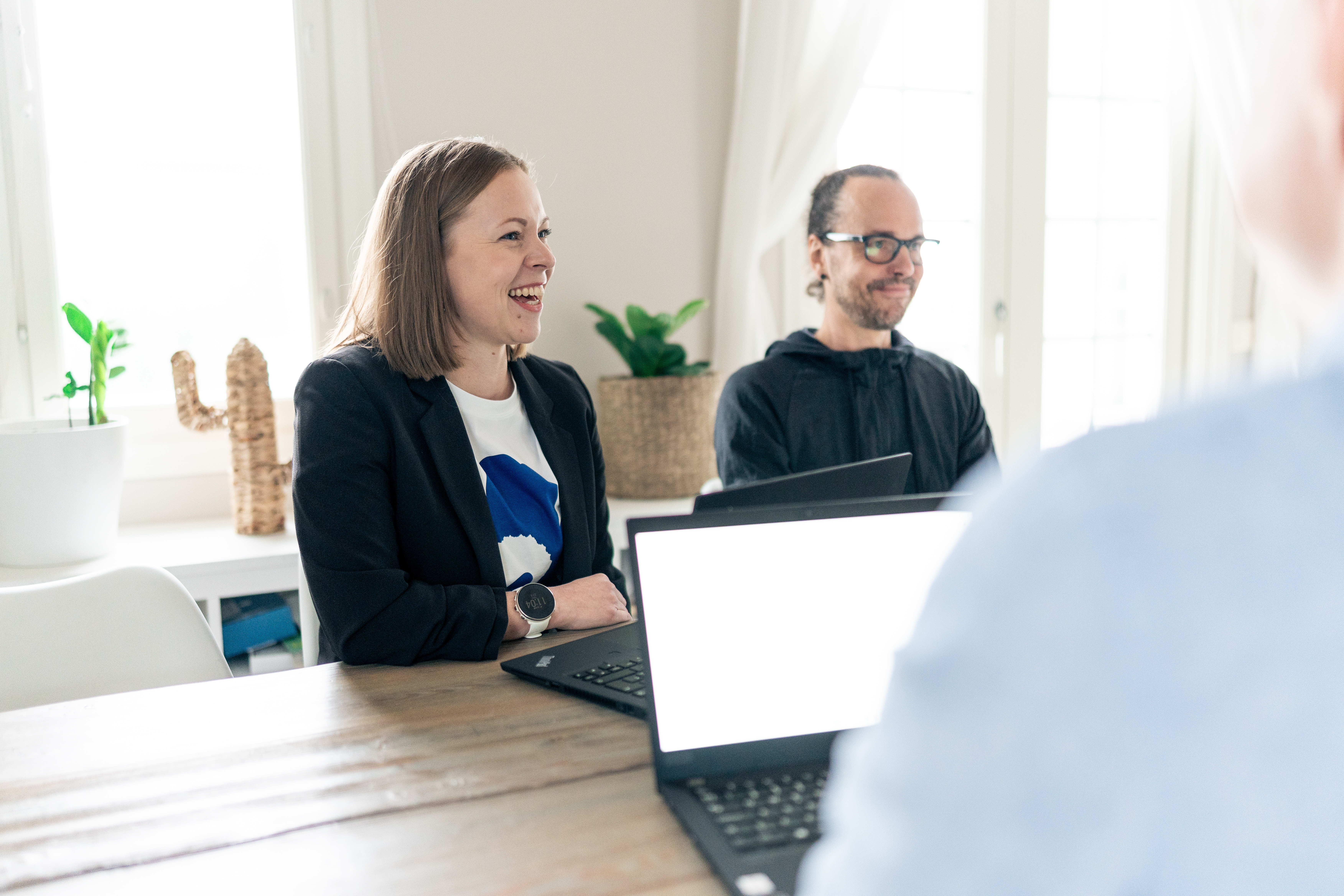
Imua rekryihin – kuinka lisätä tilitoimistotyön houkuttelevuutta?
"Neljän päivän työt yhdessä päivässä"
Digiloikka tapahtunut Fennoan käytön myötä, olen tosi tyytyväinen. Yhtenä konkreettisena esimerkkinä ajansäästöstä, ennen neljä päivää aikaa vieneen asiakkaan tekee nykyisin Fennoassa yhdessä päivässä.
Milja Saari, Tilitoimisto MilTilil Oy
"Muuta vaihtoehtoa ei oikeasti olekaan."
Fennoa täyttää sähköisen maailman vaatimukset.
Jukka Lindfors, Dito Yrityspalvelut Oy
”Ihmisläheistä palvelua”
Fennoa vastaa haasteeseen – käyttäjää ajatellen ja kuunnellen. Nopeaa ja ihmisläheistä palvelua.
Juhani Saukkonen, Tilitoimisto Todacon
”Tehokas työväline”
Kätevä Fennoa, asiakkaalle hyvä kokonaisuus ja tekijälle tehokas työväline.
Susanna Arola
”Kun ohjelma on selkeä ja yksinkertainen asiakkaille, se vähentää meidän työmääräämme”
Fennoan käyttöliittymä on todella helposti ymmärrettävä. Jo siitä hetkestä lähtien, kun Fennoaan kirjautuu sisään, se eroaa muista ohjelmistoista selkeydellään ja maalaisjärjellään.
Erja Hipari-Rantala ja Matti Rantala, Tilitoimisto Primelaskenta
”Heti kun tutustuin Fennoa-ohjelmistoon, ajatukseni oli, että tämä se on.”
Fennoan käyttö on selkeää, jos vähääkään ymmärtää taloushallinnosta. Sen käytössä pääsee helposti alkuun eikä se vie paljon aikaa.
Merja Lindfors, Meritili Oy
”Fennoa avaa mahdollisuuksia niin tilitoimistolle kuin sen asiakkaille.”
Fennoa on meille tärkeä kumppani ja saamme olla mukana kehittämässä järjestelmää yhdessä. Hyvää palvelua ilman turhaa byrokratiaa!
Johan Rönnqvist, Jbb
”Toimii kuin ajatus”
Fennoan käytettävyys on omaa luokkaansa.
Pasi Tilander, Economer Oy
”Saan nyt kaikki kätevästi saman katon alta. Kaiken kaikkiaan tämä on win-win-tilanne minulle ja tilitoimistolleni.”
Enää ei tarvitse pyöritellä papereita läheskään niin paljon kuin aiemmin – eikä kirjanpitäjän tarvitse pyöritellä päätään ostolaskujen kanssa, kun ne kaikki tulevat nykyään helpossa muodossa sähköisesti.
Timo Vainio, VeViTek Oy
”Parempaa palvelua asiakkaillemme”
Otimme Fennoan käyttöön viime syksynä. Huomasimme heti, että tämähän on todella helppokäyttöinen palvelu verrattuna muihin ohjelmistoihin. Kaikki loksahti heti kohdilleen.
Jaana Salo
Rakkaudesta taloushallintoon
Me Fennoalla rakennamme taloushallinnon tulevaisuutta. Yli 200 vuoden yhteinen kokemuksemme alalta on rakennettu suoraan ohjelmistoon, jotta se palvelee tilitoimistojen arkea mahdollisimman hyvin.
Olemme kotimainen toimija, jonka palvelimet sijaitsevat Suomessa ja päätökset sekä kehitys tehdään lähellä sinua.
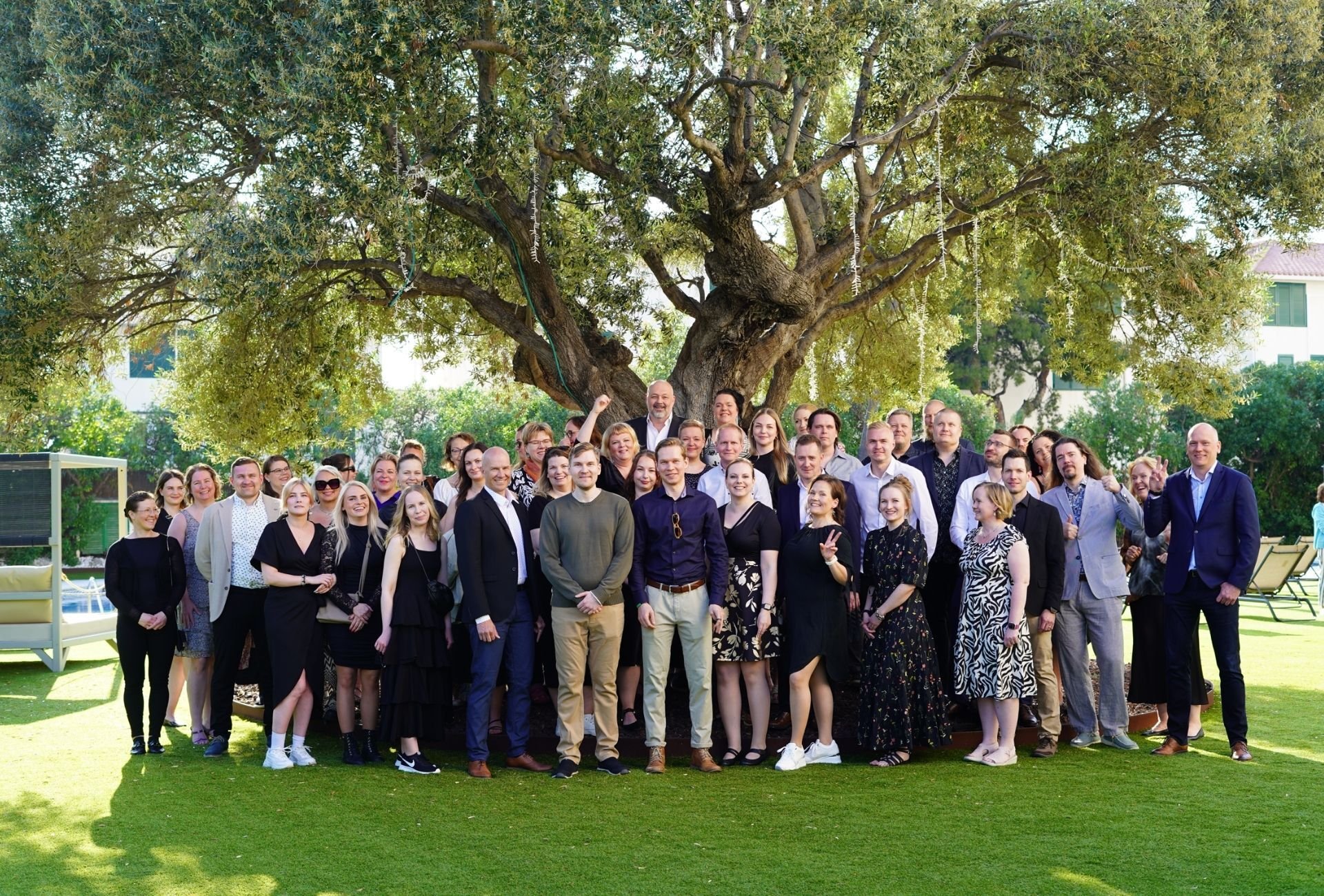